Integrating Reanimated
Yes! TrueSheet
does support react-native-reanimated. One use case would be a floating action button (FAB) to the sheet's movement, adjusting its position, opacity, or scale as the Sheet is being dragged.
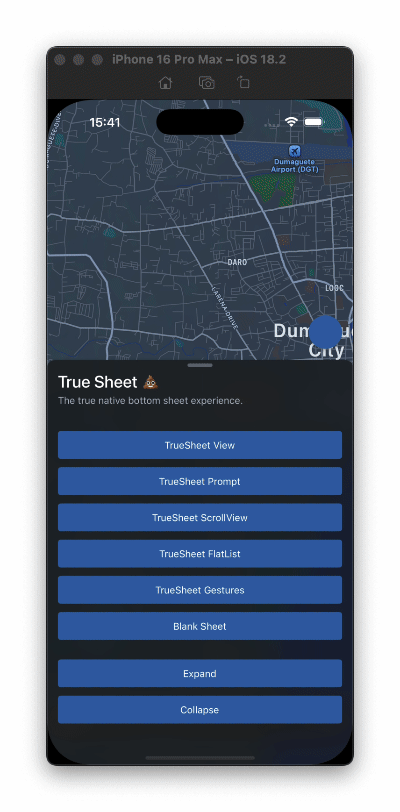
How?
In this example, we will use the onDragChange
event to listen to size changes while dragging.
You can checkout example for the complete implementation.
1. Define a handler hook
import { useEvent, useHandler } from 'react-native-reanimated'
type DragChangeHandler = (sizeInfo: SizeInfo, context: unknown) => void
export const useDragChangeHandler = (
handler: DragChangeHandler,
dependencies: DependencyList = []
) => {
const handlers = {
onDragChange: handler,
}
const { context, doDependenciesDiffer } = useHandler(handlers, dependencies)
return useEvent<DragChangeEvent>(
(event) => {
'worklet'
const { onDragChange } = handlers
if (onDragChange && event.eventName.endsWith('onDragChange')) {
onDragChange(event, context)
}
},
['onDragChange'],
doDependenciesDiffer
)
}
2. Attach the event handler
import Animated, { useSharedValue, useAnimatedStyle } from 'react-native-reanimated'
const AnimatedButton = Animated.createAnimatedComponent(TouchableOpacity)
const AnimatedTrueSheet = Animated.createAnimatedComponent(TrueSheet)
const App = () => {
const buttonY = useSharedValue(0)
const dragChangeHandler = useDragChangeHandler((sizeInfo: SizeInfo) => {
'worklet'
buttonY.value = -sizeInfo.value
})
const $animatedStyle: ViewStyle = useAnimatedStyle(() => ({
transform: [{ translateY: buttonY.value }],
}))
return (
<View>
<AnimatedButton style={$animatedStyle} />
<AnimatedTrueSheet
sizes={['auto', '69%', 'large']}
onDragChange={dragChangeHandler}
>
<View />
</AnimatedTrueSheet>
</View>
)
}