Resizing Programmatically
TrueSheet
has a main prop called sizes
which allows you to define the sizes that the sheet can support. This is an array of SheetSize
that supports sizes like "auto"
, "medium"
, "large"
, etc.
In some cases, you may want to resize the sheet programmatically for a better experience.
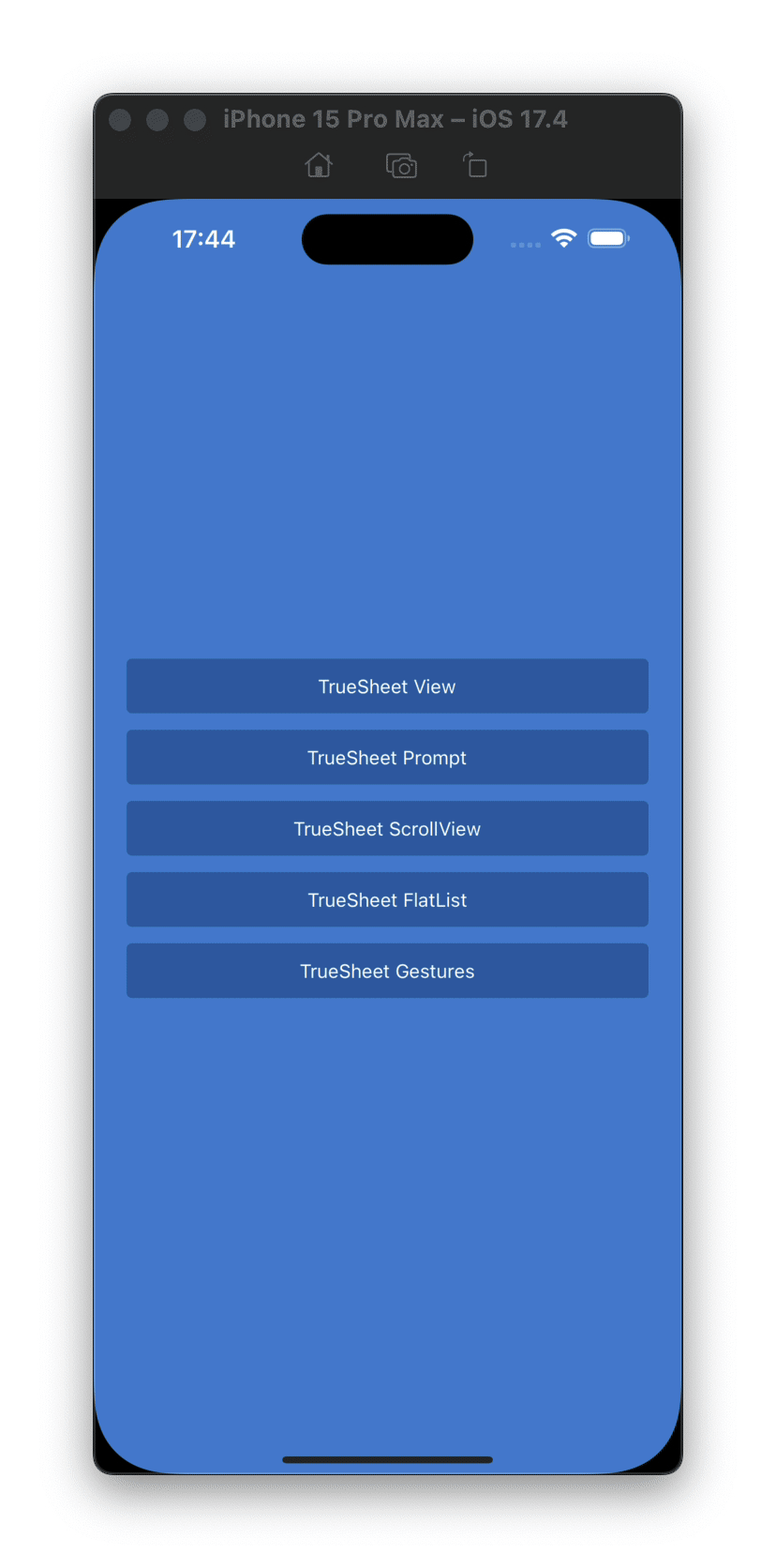
How?
Resize Programmatically
Define the sheet and use the resize
method.
const App = () => {
const sheet = useRef<TrueSheet>(null)
const resize = async () => {
// Resize to 69%
await sheet.current?.resize(1)
console.log('Yay, we are now at 69% 💦')
}
return (
<TrueSheet name="resizing-sheet" ref={sheet} sizes={['auto', '69%', 'large']}>
<Button onPress={resize} title="Resize" />
</TrueSheet>
)
}
tip
You can also do it globally using the related global method.
TrueSheet.resize('resizing-sheet', 1)
info
sizes
can only support up to 3 sizes. collapsed, half-expanded, and expanded.
Listening to Size Change
For some reason, if you want to get the resolved size, you can listen to size changes by providing the onSizeChange
event.
The event comes with the SizeInfo
that you can use to get the size value
.
const App = () => {
const sheet = useRef<TrueSheet>(null)
const resize = async () => {
// Resize to 69%
await sheet.current?.resize(1)
}
const handleSizeChange = (e: SizeChangeEvent) => {
console.log(e.nativeEvent.index, e.nativeEvent.value) // Do whatever you need from this size ✅
}
return (
<TrueSheet
ref={sheet}
sizes={['auto', '69%', 'large']}
onSizeChange={handleSizeChange}
>
<Button onPress={resize} title="Resize" />
</TrueSheet>
)
}
info
The event will also trigger when the user drags the sheet into a size.